Harry
·
25 Apr 2023
·
1 min read
How to solve the Stock Span problem in Javascript
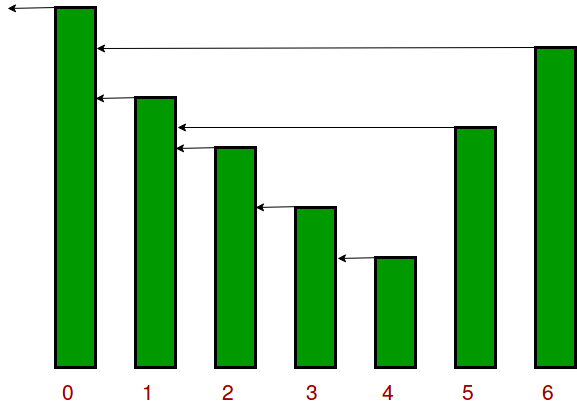
The Stock Span problem is a popular financial problem commonly known among the CS community
Stock Span is defined as a number of consecutive days prior to the current day when the price of a stock was less than or equal to the price at current day.
Like in most problems, the Stock Span problem can be solved by using different algorithms and data structures —including brute force implementations.
In this post, I'm going to show you how to solve it efficiently with a time complexity of O(n)
, using a Stack as a data structure in Javascript.
Given a list of prices of a single stock for N number of days, find the stock span for each day.
function stockSpan(prices) {
const stack = [0],
span = [1]
for (let i = 1; i < prices.length; i++) {
while (stack.length && prices[stack[stack.length - 1]] < prices[i]) {
stack.pop()
}
if (prices[stack[stack.length - 1]] > prices[i]) {
span[i] = i - stack[stack.length - 1]
}
stack.push(i)
}
return span
}
For a given input like:
stockSpan([100, 60, 70, 65, 80, 85])
The output should be:
[1, 1, 2, 1, 4, 5]